Authentication
Learn how to authenticate your requests to the Cosmic API.
Get your API keys
Cosmic uses API keys to authenticate requests. For the following examples, you will need your:
- Bucket slug
- Bucket read key
- Bucket write key
You can get your API access keys by going to Bucket Settings > API Access in the Cosmic dashboard.
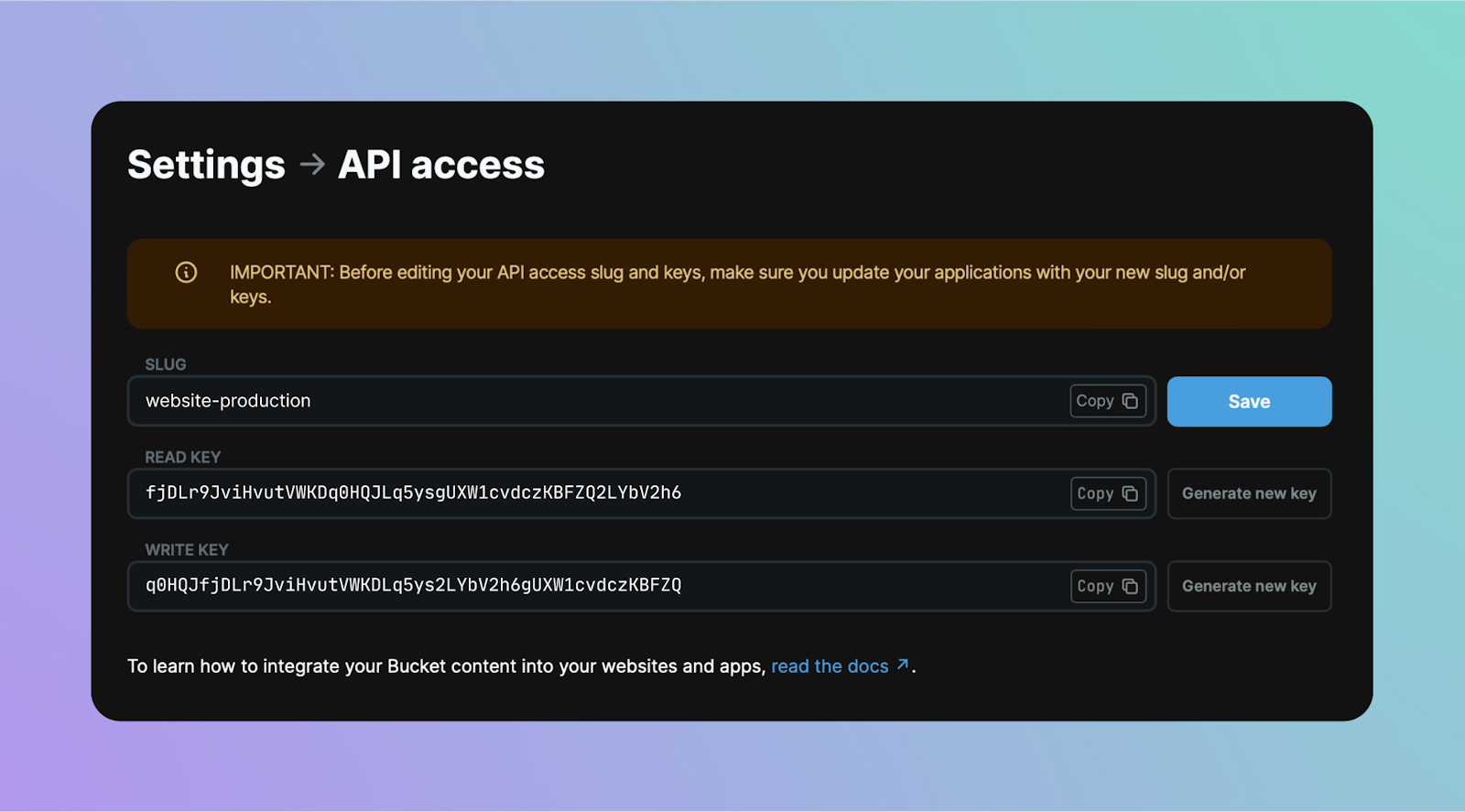
Use your API keys
Use the methods below to use your Cosmic API keys.
Before you can make requests to the Cosmic API, you will need to grab your Bucket slug and API keys from your dashboard. Find them in Bucket Settings » API Access.
GET
/v3/buckets/:bucket_slug/objects// Import
import { createBucketClient } from '@cosmicjs/sdk';
// Authenticate
const cosmic = createBucketClient({
bucketSlug: 'BUCKET_SLUG',
readKey: 'BUCKET_READ_KEY',
writeKey: 'BUCKET_WRITE_KEY',
});
// Fetch content
await cosmic.objects
.find({
type: 'posts',
})
.limit(1);
// Write content
await cosmic.objects.insertOne({
title: 'Blog Post Title',
type: 'posts',
metadata: {
content: 'Here is the blog post content...',
seo_description: 'This is the blog post SEO description.',
featured_post: true,
},
});
Never expose your Bucket write key in any client-side code.