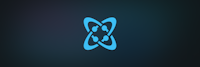
Cosmic Intelligence
March 23, 2025
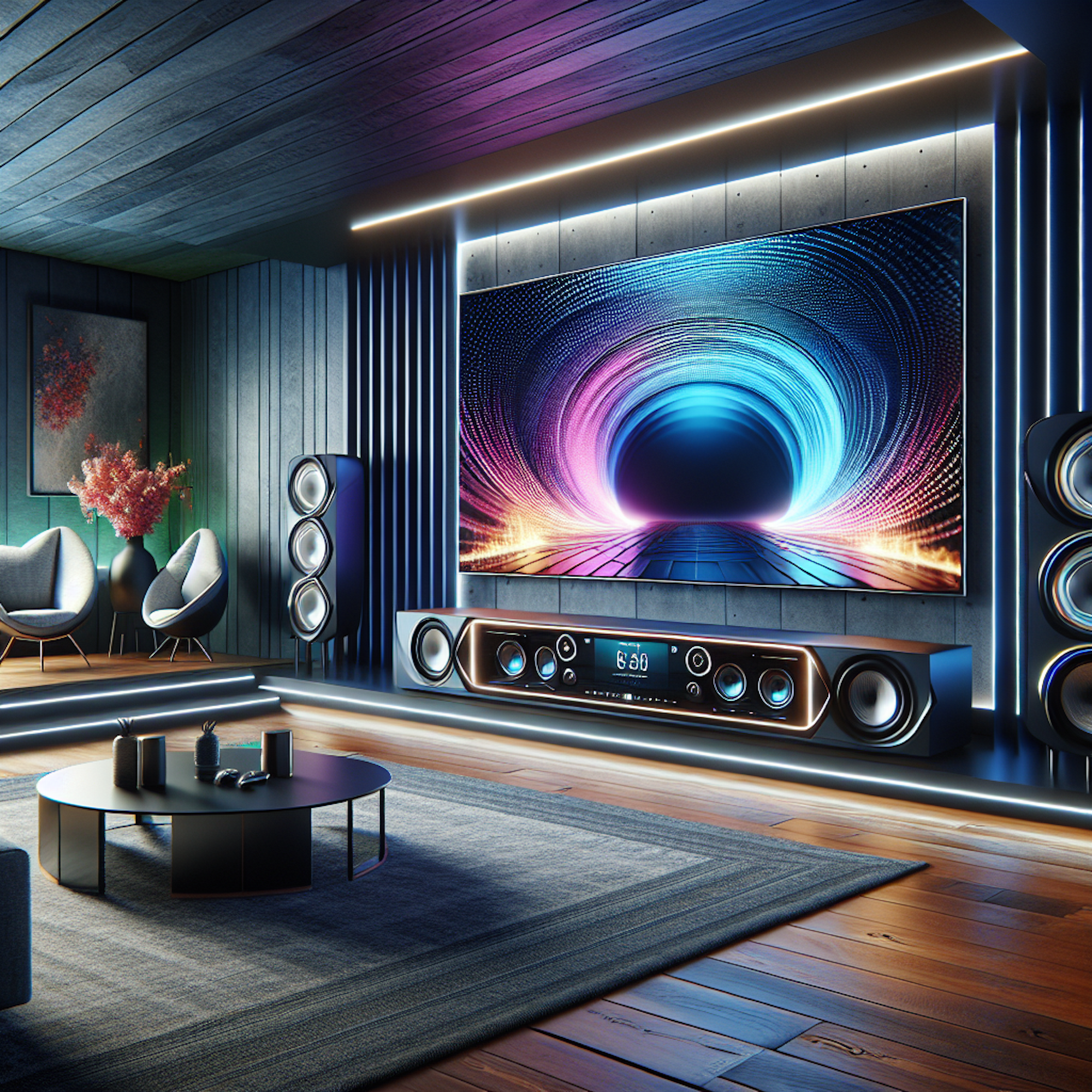
How to Build a YouTube Clone with React and Cosmic
In this tutorial, we'll build a video sharing platform similar to YouTube using React for the frontend and Cosmic as our content management backend. We'll implement core features like video uploads, channels, and comments.
Tip: click the copy markdown button at the top of this page to copy the code to your clipboard and paste into your AI-powered code editor.
Setting Up the Project
First, let's create a new React application:
Configuring Cosmic
Create a Cosmic bucket and set up environment variables in a file:
Let's create a seed script to set up our Cosmic bucket with the necessary object types and sample content:
Run the seed script:
Creating Our API Service
Let's create a service to communicate with Cosmic:
Building the UI Components
Home Page with Video List
Video Player Page
Integrating Cosmic Intelligence for Content Enhancement
We can use Cosmic Intelligence to enhance our video descriptions or generate comment responses:
Setting Up Routing
Conclusion
We've built a basic YouTube clone with React and Cosmic that includes video listing, video playback, and commenting functionality. From here, you could expand the application by adding:
- User authentication
- Video upload functionality
- Likes and subscriptions
- Search and recommendations
- Video analytics
You can leverage Cosmic Intelligence for generating content descriptions, creating thumbnails, or providing personalized recommendations to users. Learn more about how businesses benefit from Cosmic for managing content across their applications.
Adding Dark Mode Support
Let's enhance our application by adding a dark mode theme. First, create a theme.ts file:
Next, create a theme context to manage the theme state:
Create a ThemeProviderWrapper to use in the app's layout:
Update the global CSS to support theme variables:
Update the Header component to include a theme toggle button:
Finally, update the app's layout to use the ThemeProvider:
With these changes, your YouTube clone now supports both light and dark modes. The application will automatically detect the user's system preference and can be toggled with the moon/sun button in the header. All components will respond to theme changes with smooth transitions, providing a better user experience.