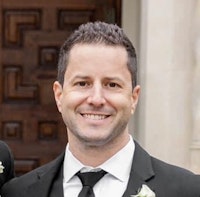
Tony Spiro
December 29, 2015
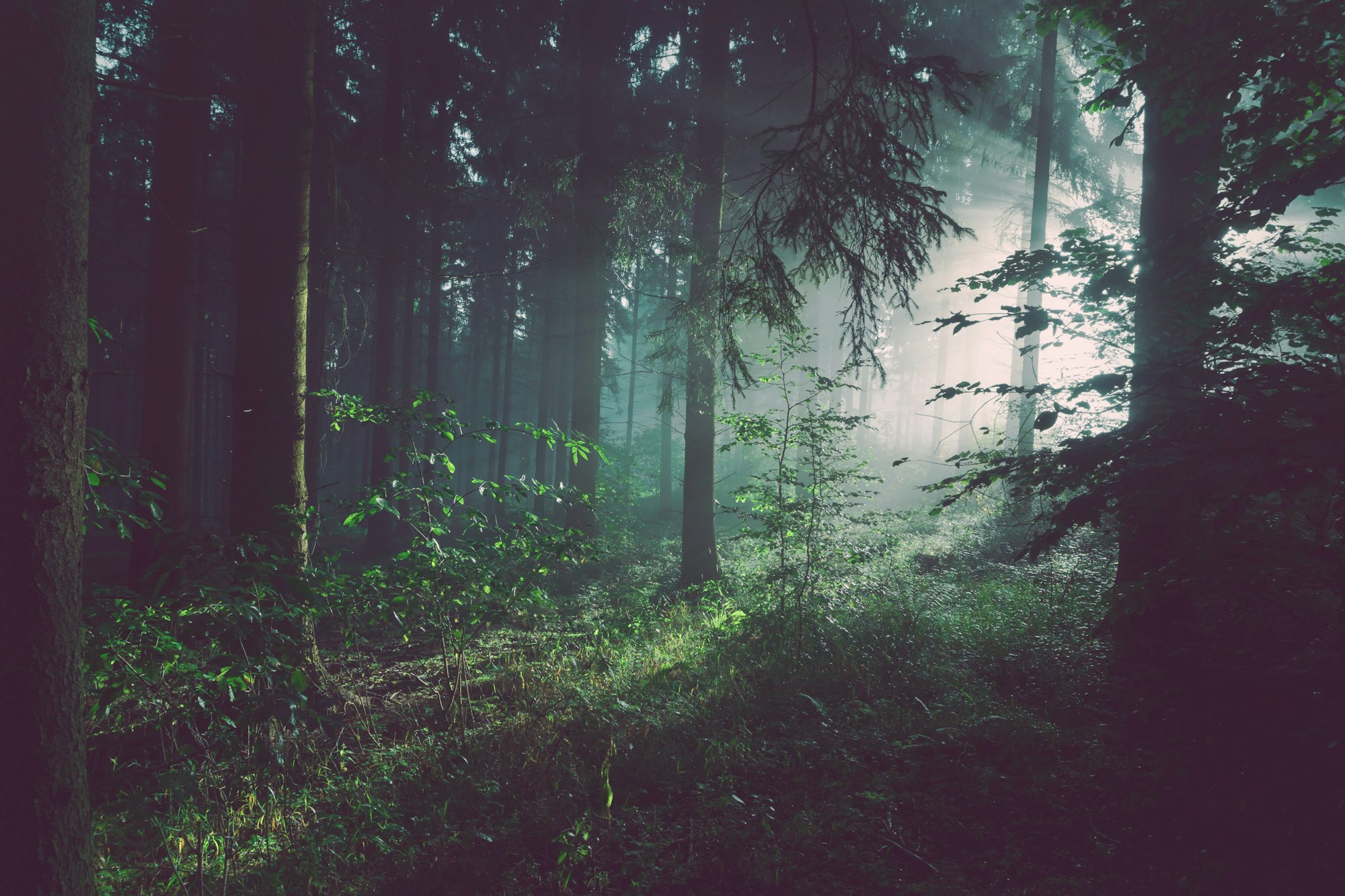
In this short tutorial I'll show you how easy it is to add a CMS to a simple browser app using the Cosmic API. It will literally take you 2 minutes to build. Our app will consist of just 3 files:
1. index.html
2. app.js
2. package.json
Let's get started shall we? In your terminal of choice run the following commands:
mkdir easy-browser-example
cd easy-browser-example
npm install cosmicjs
npm install browserify -g
Now let's build our index.html file. Run the following command in your terminal:
vim index.html
Add the following to our index.html file:
<!DOCTYPE html>
<html>
<head>
<title>Cosmic Easy Browser Example</title>
</head>
<body>
<h1 id="title">If you see this, something isn't working...</h1>
<div id="content"></div>
<div id="metafields"></div>
<script src="app.browser.js"></script>
</body>
</html>
We could just as easily use jQuery and Ajax to render our content, but for this example we will use the official Cosmic Node.js package. Now create a file called app.js:
vim app.js
And add the following to app.js:
// app.js
var Cosmic = require('cosmicjs')
const bucket = { slug: 'easy-browser-example' }
const object = { slug: 'home' }
Cosmic.getObject({ bucket }, object, (err, res) => { var object = res.object
document.getElementById('title').innerHTML = object.title
document.getElementById('content').innerHTML = object.content
document.getElementById('metafields').innerHTML = '<pre>' + JSON.stringify(object.metafields, null, 2) + '</pre>'
})
Notice that in our app.js file we are returning content from the Cosmic API, and then attaching our content to the DOM elements at "title","content" and "metafields".
Next we'll add a package.json file that will allow us to add some simple scripts to "browserify" our app.js file:
vim package.json
Add the following to a new file titled package.json:
{
"name": "easy-browser-example",
"main": "app.js",
"scripts": {
"browserify": "browserify app.js -o app.browser.js"
}
}
Now let's run our our scripts to bundle our code into the browser. Run the following script which will bundle the new file to app.browser.js:
npm run browserify
Now view the index.html file in your browser and you will see that the content from our example bucket "easy-browser-example" can be seen and the metafields data is rendered to a string to show you what data is available. Taking this a step further, you can see how powerful this can be if you add React or Angular into the mix.
I hope you enjoyed this short tutorial. If you have not already, you can sign up for a Cosmic account, and begin playing with this example using content from your own bucket. Thanks and happy building!