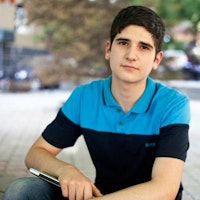
Hayk Adamyan
February 15, 2018
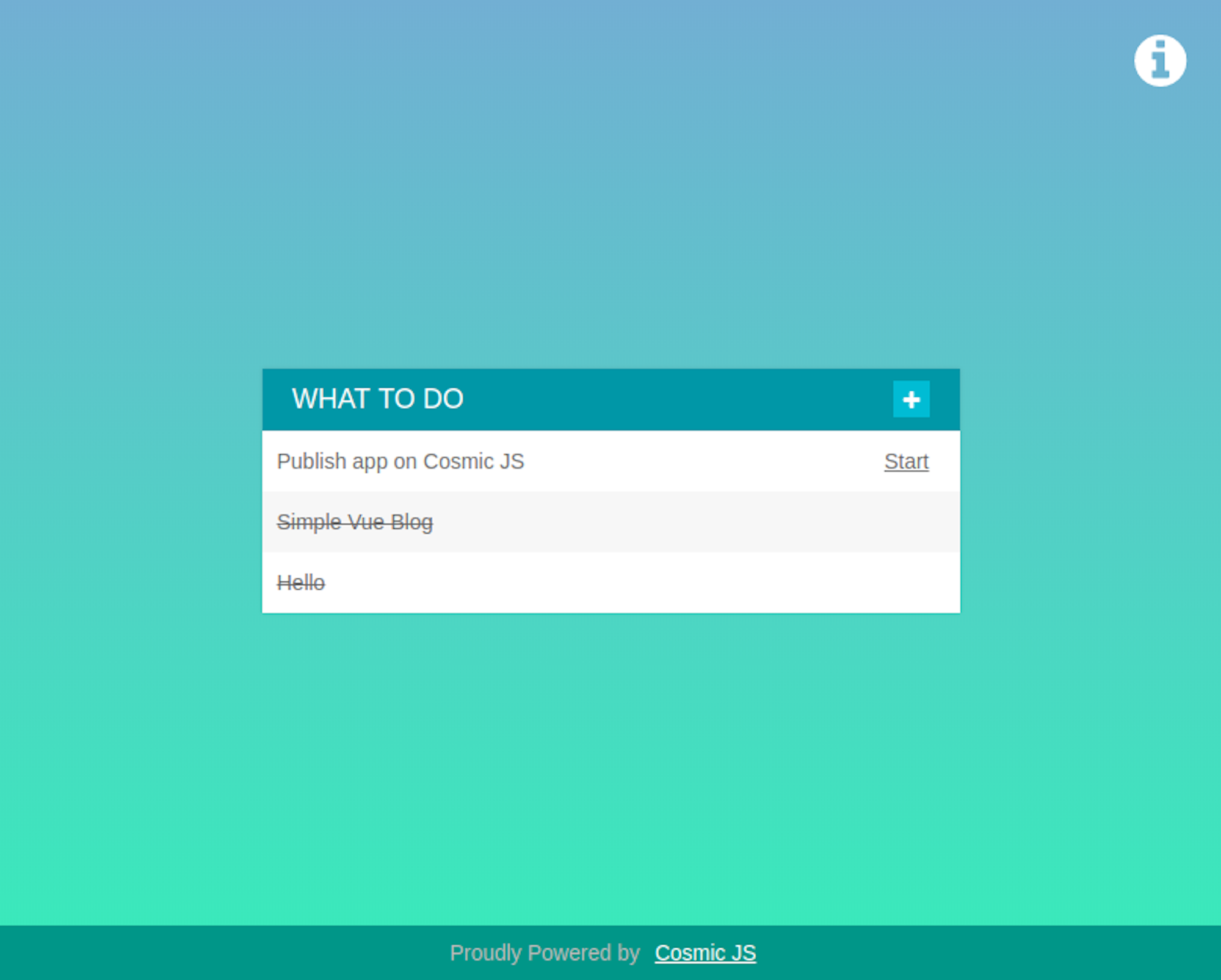
In this tutorial, I'm going to show you how to create a Todo list app with pomodort timer included using React and Cosmic. We will be using Cosmic npm package for implementing the basic CRUD for our objects and to retrieve data and media from Cosmic. Let's get started.
TL;DR
View the Demo
Download the GitHub repo
Prerequisites
You will be requiring to install Node JS and npm before starting. Make sure you already have them.
Getting Started
Doing everything using the existing git repo
First of all, you have to be sure you have node > 6.x and yarn installed, than run the following commands:
After successfull completion of the last command browser window will automatically open package.json
will look like this.
Now the app has to be running on http://localhost:300
Let's setup webpack.config.js
Code written here is responsible for compiling React JS.
Setting up ./src/components/app/app.js
In this file we are creating the home page file. So in state we are keeping the following data.
- Form-> title : Responsible for new task input value
- List: Array of strings, here are saved all the tasks
- showNewTaskInput: Boolean value, responsible for show/hide the new task input
- notification: Current notification name, we also have types for notifications listed below as an object, here are sorted the names for the notification types.
We use the following methods in our Class
:
- Constructor: We are setupping new
Api service
, this gonna be used to connect to the Cosmic API. - ComponentWillMount: This is function which belongs to React component lifecycle, here we just call another method created by us.
- getData: Function which does a call to the API and updates the list of tasks, after what we reset the value of new task input using
this.resetForm()
- createNotification: Function which gets a notification type as an argument and puts it in the state for further use
- onChange: Function is responsible to keep the new task title in the state.
- onSave: Function creates a new task using the Cosmic api.
- onStart: Function is responsible for starting the timer for specific task, it calls the Api to save the start time in the Cosmic's db.
- onDone: Function is responsible for setting the status of specific task to "Done".
- onUpdate: Function is responsible to update specific task's title.
- showNewTaskInput: Function shows new task input.
- resetForm: Function is responsible for cleaning the value of the new task input.
- render: In render we put our html, and call our functions mentioned above.
Setting up ./src/task-list/list-item/index.js
Inlist-item
we are creating each task item
In state we are keeping 2 items:
- editing: If the title is currently in process of change
- title: Current title of the task
Below we 3 functions coming after. They will call the functions passed to component by props
.
Next 2 functions have some logic in them. Here they are:
- onEdit: Function which is responsive for updating the state
editing
item. - onChange: Function which changes the
title
item in the state.
Then we have 2 React component lifecycle methods:
- componentWillReceiveProps: In this function we are checking if component has recieved new props we are updating our local state.
- render: All the HTML code goes here. Where we call our functions mentioned above.
Setting up ./src/components/countdown/index.js
In this file we are using Moment JS for doing basic calculation for the time countdown.
We are all set up, you can run the application, and enjoy it.